Full SDK Integration
Stop users ever leaving your dApp in just a few lines of code. Let’s get cracking.
How to use our full SDK
For the best user experience and maximize your conversions for all users.
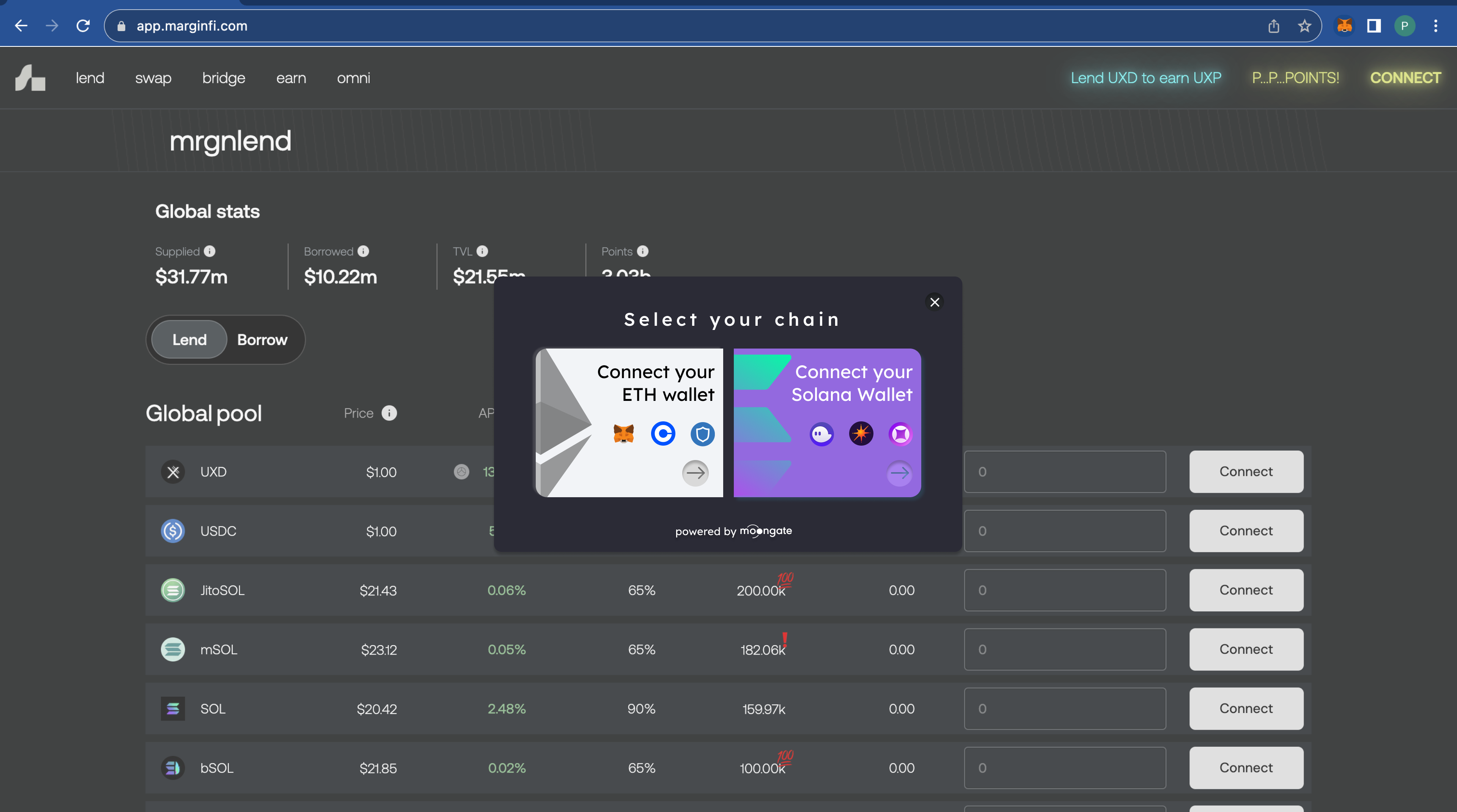
This is what your user will see once they press the connect wallet button.
Requirements
Take-off Safety Procedures Before we accelerate, let’s check if you’re ready to go. Please ensure you are using the Solana Labs Wallet adapter in your dApp. - This SDK is built for React based projects only. If you are looking to implement using another stack, talk to our staff on Discord. - If you are using a custom wallet adapter instead of the Solana Labs Wallet Adapter - speak to a member of our staff on Discord and we will custom cater our integration for you.
How to Integrate full SDK:
Alright, we’re ready to start integrating!
Step 1
On your frontend, install the dependencies using npm.
npm i @moongate/google-button @moongate/moongate-adapter
Step 2
Navigate to your Solana Labs wallet adapter where the wallets are defined.
It should look something like this:
const wallets = useMemo(
() => [new UnsafeBurnerWalletAdapter()],
// eslint-disable-next-line react-hooks/exhaustive-deps
[network]
);
Then, import the wallet adapter from the top of the file like follows:
import { registerMoonGateWallet } from "@moongate/moongate-adapter";
Next, instantiate the wallet adapter within the wallets declaration like follows:
registerMoonGateWallet({ authMode: "Ethereum", position: "top-left", logoDataUri: "OPTIONAL ADD IN-WALLET LOGO URL HERE", buttonLogoUri: "ADD OPTIONAL LOGO FOR WIDGET BUTTON HERE" })
registerMoonGateWallet({ authMode: "Google", position: "top-right", logoDataUri: "OPTIONAL ADD IN-WALLET LOGO URL HERE", buttonLogoUri: "ADD OPTIONAL LOGO FOR WIDGET BUTTON HERE" })
registerMoonGateWallet({ authMode: "Twitter", position: "top-right", logoDataUri: "OPTIONAL ADD IN-WALLET LOGO URL HERE", buttonLogoUri: "ADD OPTIONAL LOGO FOR WIDGET BUTTON HERE" })
registerMoonGateWallet({ authMode: "Apple", position: "top-right", logoDataUri: "OPTIONAL ADD IN-WALLET LOGO URL HERE", buttonLogoUri: "ADD OPTIONAL LOGO FOR WIDGET BUTTON HERE" })
// just above the wallets memo
const wallets = useMemo(
() => [
new UnsafeBurnerWalletAdapter(),
],
[network]
);
Step 3
Navigate to where your Wallet Adapter Connection button is defined.
It is usually called WalletMultiButton - or similar.
<WalletMultiButton /> // this is what it usually looks like
At the top of where this button is defined, call the following imports
import { Button } from "@moongate/google-button";
import {
WalletMultiButton,
WalletDisconnectButton,
useWalletModal,
} from "@solana/wallet-adapter-react-ui";
import { useWallet } from "@solana/wallet-adapter-react";
Next, add the following hooks to the main function component of where you want to define the button.
const { select, connected } = useWallet();
const { visible, setVisible } = useWalletModal();
Then, replace the existing button with this:
<Button select={select} connected={connected} setVisible={setVisible} text="JOIN" />
That’s it. Quick and easy. You’re ready to go! That’s all it took to anti-noob your dApp.